题目一:
要求实现这样一个带功能的界面的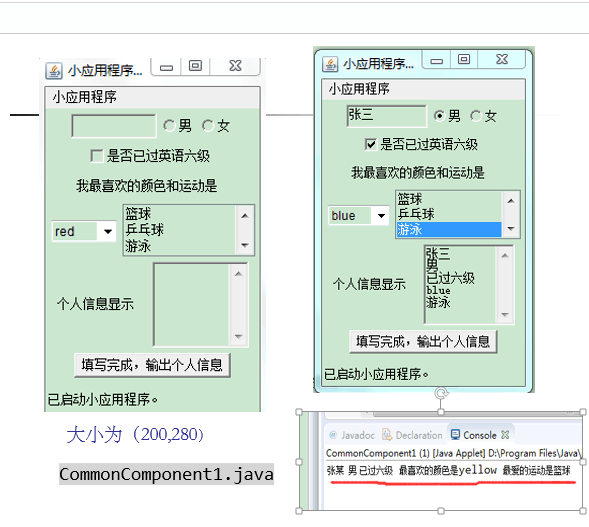
1 | package home_work; |
运行结果
题目二:
实现一个计算器1 | package home_work; |
题目三:
实现如下图所示界面(左侧为一个列表,右侧各个信息输入后,点击“增加”,列表中添加相应学生信息,若选中左侧列表中的某条信息,点击“删除”,可删除相应学生)1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113package home_work;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.ItemEvent;
import java.awt.event.ItemListener;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import javax.swing.JOptionPane;
import javax.swing.WindowConstants;
public class job_2 {
Frame f;
Panel p1, p2;
List list;
Label label_1, label_2;
TextField text;
Button button1, button2;
CheckboxGroup cg;
Checkbox boy, girl;
Choice choice;
int select = 0;
public job_2() {
f = new Frame("java课学生信息输入窗口");
f.setLayout(new BorderLayout());
f.setLocation(700, 300);
// f.setSize(200, 200);
f.setVisible(true);
p1 = new Panel();
list = new List(10, false);
list.add("姓名 性别 班级");
p1.add(list);
f.add(p1, BorderLayout.WEST);
p1 = new Panel();
p1.setLayout(new FlowLayout());
label_1 = new Label("姓名");
text = new TextField(5);
label_2 = new Label("性别");
cg = new CheckboxGroup();
boy = new Checkbox("男", cg, true);
girl = new Checkbox("女", cg, false);
choice = new Choice();
choice.add("信算系");
choice.add("数学系");
button1 = new Button("增加");
button2 = new Button("删除");
p1.add(label_1);
p1.add(text);
p1.add(label_2);
p1.add(boy);
p1.add(girl);
p1.add(choice);
p1.add(button1);
p1.add(button2);
f.add(p1, BorderLayout.EAST);
button1.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(text.getText().length() < 1) {
JOptionPane.showMessageDialog(null, "请输入姓名!");
}else {
list.add(
String.valueOf(text.getText() + " "
+ cg.getSelectedCheckbox().getLabel()+ " "
+ choice.getSelectedItem() + " ")
);
}
}
});
list.addItemListener(new ItemListener() {
public void itemStateChanged(ItemEvent e) {
select = list.getSelectedIndex();
}
});
button2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
if(select != 0) {
list.remove(select);
}
}
});
f.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {
f.dispose();
System.exit(0);
}
});
}
public static void main(String[] args) {
new job_2();
}
}
运行结果